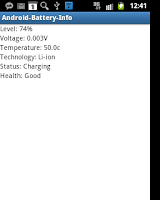
Untuk membuat aplikasi ini butuh permission BATTERY_STATS yang menunjukkan bahwa aplikasi boleh melihat status baterai milik perangkat Android.
Caranya buat project baru, kemudian buat tampilan sederhana dengan 5 buah TextView pada file layout activity_main.xml seperti ini :
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/LinearLayout1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<TextView
android:id="@+id/tvBatteryLevel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Battery Level" />
<TextView
android:id="@+id/tvBatteryVoltage"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Battery Voltage" />
<TextView
android:id="@+id/tvBatteryTemperature"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Battery Temperature" />
<TextView
android:id="@+id/tvBatteryTechnology"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Battery Technology" />
<TextView
android:id="@+id/tvBatteryStatus"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Battery Status" />
<TextView
android:id="@+id/tvBatteryHealth"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Battery Health" />
</LinearLayout>
Kemudian pada kode program utama di MainActivity.java ketikkan seperti ini :
package com.amijaya.android_battery_info;
import android.os.BatteryManager;
import android.os.Bundle;
import android.app.Activity;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.content.IntentFilter;
import android.view.Menu;
import android.widget.EditText;
import android.widget.TextView;
// http://cariprogram.blogspot.com
// nuramijaya@gmail.com
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
registerReceiver(new BroadcastReceiver() {
@Override
public void onReceive(Context arg0, Intent arg1) {
// TODO Auto-generated method stub
if (arg1.getAction().equals(Intent.ACTION_BATTERY_CHANGED)){
TextView tvBatteryLevel = (TextView)findViewById(R.id.tvBatteryLevel);
TextView tvBatteryVoltage = (TextView)findViewById(R.id.tvBatteryVoltage);
TextView tvBatteryTemperature = (TextView)findViewById(R.id.tvBatteryTemperature);
TextView tvBatteryTechnology = (TextView)findViewById(R.id.tvBatteryTechnology);
TextView tvBatteryStatus = (TextView)findViewById(R.id.tvBatteryStatus);
TextView tvBatteryHealth = (TextView)findViewById(R.id.tvBatteryHealth);
tvBatteryLevel.setText("Level: "
+ String.valueOf(arg1.getIntExtra("level", 0)) + "%");
tvBatteryVoltage.setText("Voltage: "
+ String.valueOf((float)arg1.getIntExtra("voltage", 0)/1000) + "V");
tvBatteryTemperature.setText("Temperature: "
+ String.valueOf((float)arg1.getIntExtra("temperature", 0)/10) + "c");
tvBatteryTechnology.setText("Technology: " + arg1.getStringExtra("technology"));
int status = arg1.getIntExtra("status", BatteryManager.BATTERY_STATUS_UNKNOWN);
String strStatus;
if (status == BatteryManager.BATTERY_STATUS_CHARGING){
strStatus = "Charging";
} else if (status == BatteryManager.BATTERY_STATUS_DISCHARGING){
strStatus = "Dis-charging";
} else if (status == BatteryManager.BATTERY_STATUS_NOT_CHARGING){
strStatus = "Not charging";
} else if (status == BatteryManager.BATTERY_STATUS_FULL){
strStatus = "Full";
} else {
strStatus = "Unknown";
}
tvBatteryStatus.setText("Status: " + strStatus);
int health = arg1.getIntExtra("health", BatteryManager.BATTERY_HEALTH_UNKNOWN);
String strHealth;
if (health == BatteryManager.BATTERY_HEALTH_GOOD){
strHealth = "Good";
} else if (health == BatteryManager.BATTERY_HEALTH_OVERHEAT){
strHealth = "Over Heat";
} else if (health == BatteryManager.BATTERY_HEALTH_DEAD){
strHealth = "Dead";
} else if (health == BatteryManager.BATTERY_HEALTH_OVER_VOLTAGE){
strHealth = "Over Voltage";
} else if (health == BatteryManager.BATTERY_HEALTH_UNSPECIFIED_FAILURE){
strHealth = "Unspecified Failure";
} else{
strHealth = "Unknown";
}
tvBatteryHealth.setText("Health: " + strHealth);
}
}
}, new IntentFilter(Intent.ACTION_BATTERY_CHANGED));
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
}
Terakhir jangan lupa memberi permission BATTERY_STATS
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.amijaya.android_battery_info"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="16" />
<uses-permission android:name="android.permission.BATTERY_STATS"/>
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name="com.amijaya.android_battery_info.MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Jika dijalankan hasilnya :
Project androidnya bisa didownload. Jika kesulitan silakan cek caranya.
Update Contact :
No Wa/Telepon (puat) : 085267792168
No Wa/Telepon (fajar) : 085369237896
Email : Fajarudinsidik@gmail.com
No Wa/Telepon (puat) : 085267792168
No Wa/Telepon (fajar) : 085369237896
Email: Fajarudinsidik@gmail.com
atau Kirimkan Private messanger melalui email dengan klik tombol order dibawah ini :